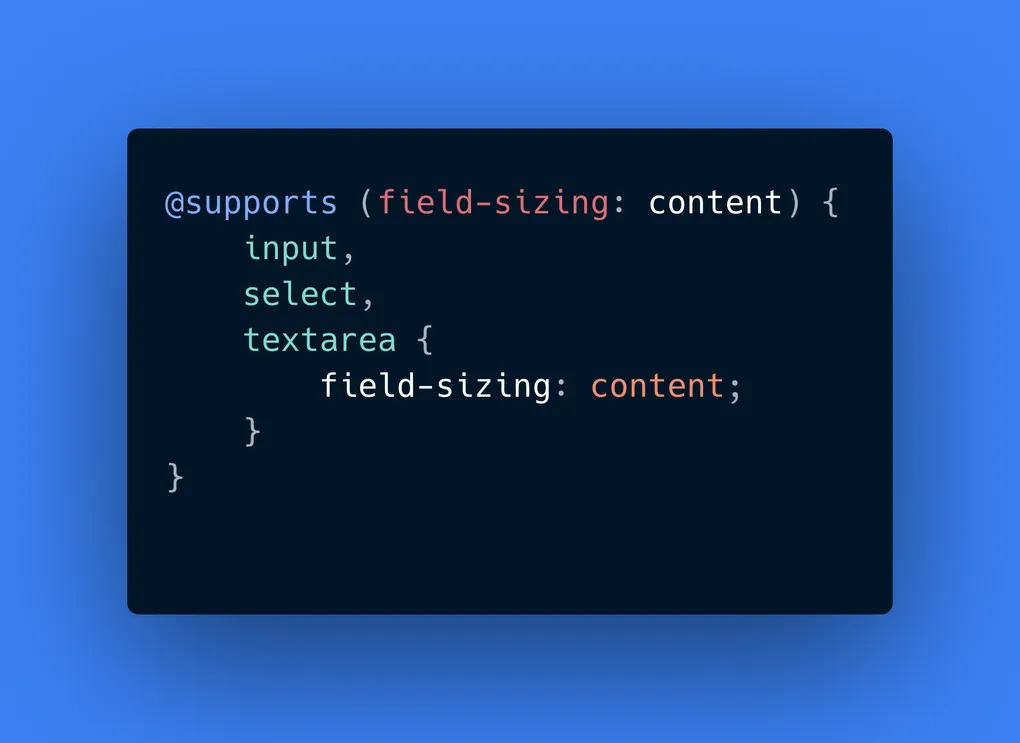
Auto-Resizing Form Fields: A Pure CSS Solution
Overcoming the Limitations of Fixed-Size Form Fields
Form fields like <input>
, <textarea>
, and <select>
are essential for
collecting user input. However, they often come with a common drawback: fixed
sizes with variable content.
When a form field’s content exceeds its fixed size, the overflow can make it difficult for users to interact with the field.
Conversely, when the content is significantly smaller than the fixed size, the field may make the interface feel unbalanced.
Setting a form field’s size often requires finding a middle ground to avoid it
being too small or too large. When dealing with a <select>
field with a static
list of options, this solution can work fairly well. For <input>
and
<textarea>
fields where the content is more dynamic, this solution can fall
apart fairly quickly.
Some elements, like <textarea>
, offer the ability to manually resize by
dragging a handle in the corner of the form field. To enable this you can set
the CSS resize
property to vertical
, horizontal
, or both
. In fact, most
browsers default this property to both
. However, this feature doesn’t work on
most mobile browsers.
The browser you are currently using doesn’t support the CSS resize
property.
As a result, the below example will not be resizable. See
https://caniuse.com/css-resize for more details.
This gives users some control, but it’s not a perfect solution. Wouldn’t it be great if all form fields could adjust automatically based on their content?
JavaScript to the Rescue
To tackle these issues, developers have often turned to JavaScript solutions
that dynamically adjust form field sizes based on content. For React developers,
packages like
React Textarea Autosize
have been a popular choice. This package provides a drop-in replacement for the
<textarea>
element that automatically resizes as the user types. At the time
of writing this post, this package has more than 11 million downloads per month.
While effective, this approach adds complexity to your codebase. Wouldn’t it be great if there was a pure CSS solution?
Enter the CSS field-sizing
Property
As of now, the field-sizing
property is relatively new and not widely
supported. Until support is more widespread, I would recommend leveraging a
@supports
rule with fallback styles, effectively making it a progressive
enhancement.
See https://caniuse.com/?search=field-sizing for more details.
CSS now offers a pure solution to auto-resize form fields. The new CSS
field-sizing
property allows form fields to adjust their size
dynamically based on their content, eliminating the need for JavaScript.
input,
select,
textarea {
field-sizing: content;
}
You are using a browser that doesn’t support the CSS field-sizing
property.
As a result, the below example will fallback to the defaults and won’t
automatically resize as its content changes.
By setting field-sizing: content
, form fields automatically resize to fit
their content. This property works with several form elements, including
<input>
, <textarea>
, and <select>
.
To maintain usability and prevent layout issues, I recommend setting minimum
and maximum size constraints on your form fields. You can achieve this using
the CSS min-inline-size
, min-block-size
, max-inline-size
, and
max-block-size
properties. These properties control the minimum and
maximum width and height of form fields, similar to min-width
,
min-height
, max-width
, and max-height
, but they adapt to different
writing modes.
Alternatively, you can use placeholders, which also impact the intrinsic
size of the fields. The length of the placeholder text determines the
initial size of the field when using field-sizing: content
.